ChargeItPro Javascript API
Payment Processing Partners, Inc. is offering a browser based payment processing solution that allows developers to easily integrate payment processing hardware into their new or existing solutions.Detailed API documentation can be found here.
Prerequisites
To get started using Easy Integrator.Download and install ChargeItProWebDriver application.
Our ChargeItProWebDriver application allows your Web Site to communicate with Hardware Devices to capture payment information and signatures.
The ChargeItProWebDriver comes pre-configured with an Emulator to allow you to test payment transactions without having hardware installed.
How to use the Emulator
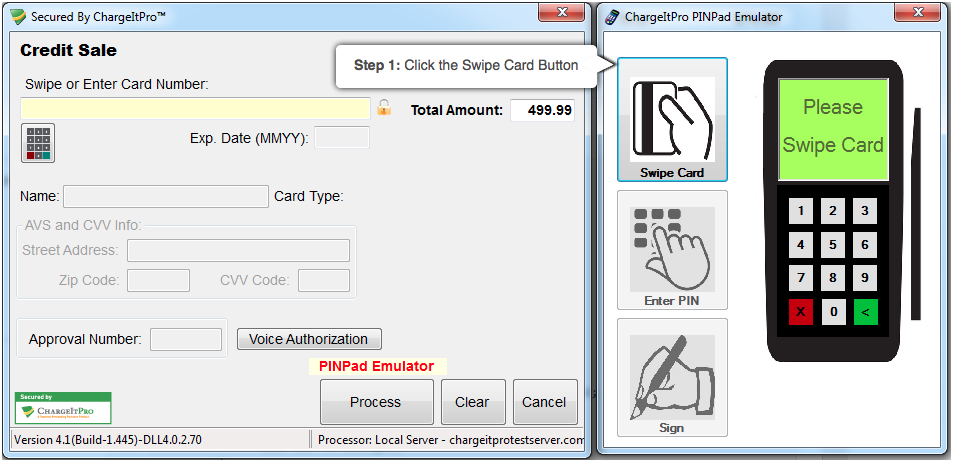
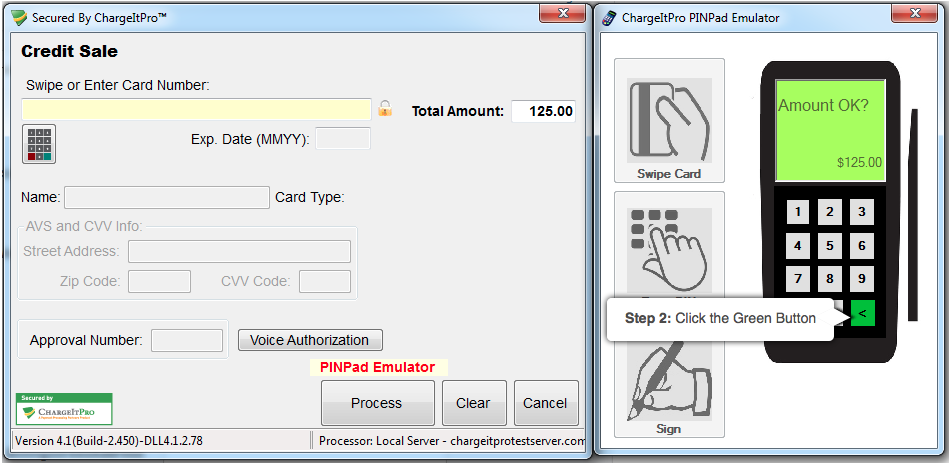
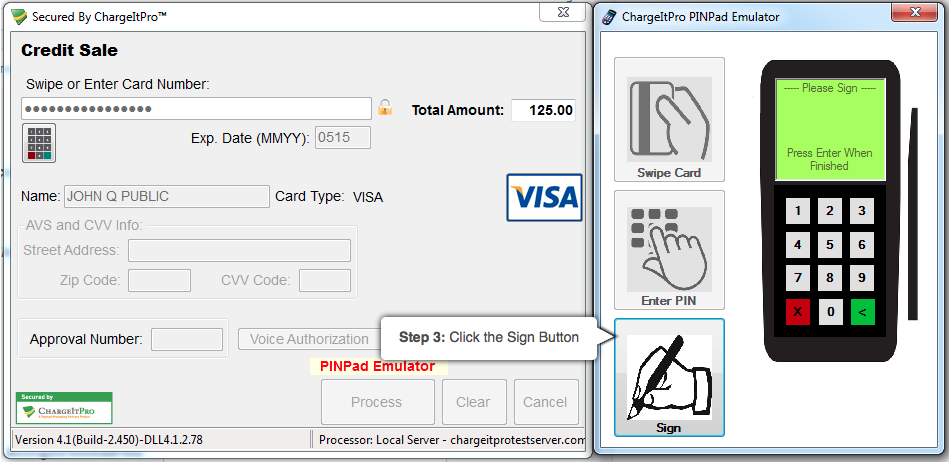
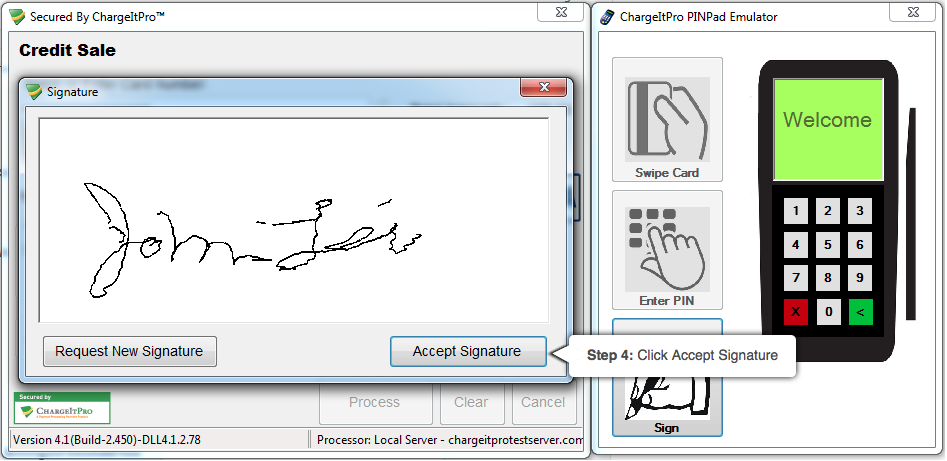
How To Setup Workstations
Authentication
All requests must be authenticated by passing in a MerchantName and MerchantKey, generated and provided by ChargeItPro.Hardware Configuration
In order for EasyIntegrator API to communicate with the hardware you will need to run setup and generate a unique ConfigurationId. This ConfigurationId must be passed on all subsequent requests.Quick Setup vs. Advanced Setup
When you run setup you will be presented with the following screen.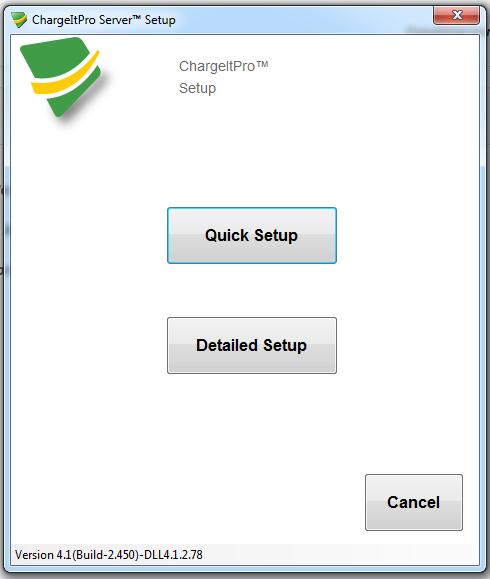
Clicking the Quick Setup button will present you with the following screen.
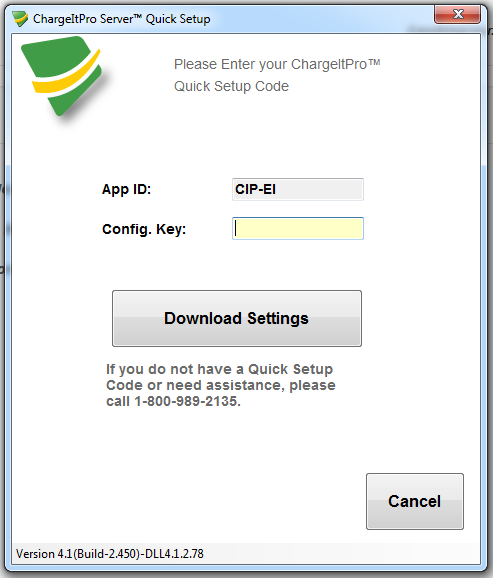
Clicking the Detailed Setup button will present you with the following screen.
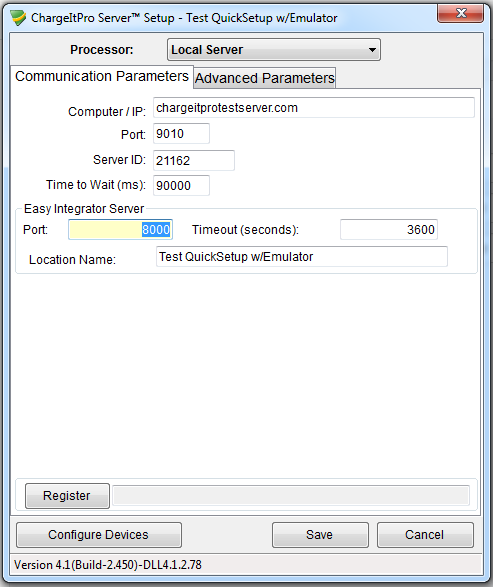
This is an example of a Setup Screen that has been integrated with EasyIntegrator that calls the RunSetup method and generates a unique ConfigurationId.
Code Example
- var request = {
- "merchantName": "DemoUser1",
- "merchantKey": "62de169ead984d709cd6acdb36d0f03e"
- };
- EasyIntegrator.runSetup(request)
- .success(function (configurationId) {
- /* IMPORTANT: You must store this configurationId */
- /* (ie in a database) and pass it in */
- /* on all Transaction Requests. */
- })
- .error(function (errorMessage) {
- alert(errorMessage);
- })
How To Process Payments
This is an example of a Demo Shopping Cart that has been integrated with EasyIntegrator.Items | Quantity | Price | |
---|---|---|---|
![]() | Blue Mixer"Every home chef needs this mixer!" -- Anson Luis, renown French Chef | $24.99 | |
![]() | Red ToasterThe perfect toaster for your kitchen. | $74.98 | |
Total | $99.97 |
Transaction Type: 

Use Tokenization
What is Tokenization?
What is Tokenization?
Description
To use Easy Integrator to process a payment transaction, create a
JSON object that contains a TransFields object
and pass it into EasyIntegrator.processTransaction.
Code Example
- var request = {
- "merchantName": "BobsRibs1",
- "merchantKey": "A3gziLB98002",
- "configurationId": "198e84701abc28da",
- "transactionType": "CreditSale",
- "transFields": {
- "amountTotal": 99.97
- }
- };
- EasyIntegrator.processTransaction(request)
- .success(function (resultsFields) {
- var transactionId = resultsFields.uniqueTransId;
- })
- .error(function (errorMessage) {
- alert(errorMessage);
- })
- .done(function () {
- });
Receipt Data
This is an example of a receipt and the fields returned from EasyIntegrator to populate the form values.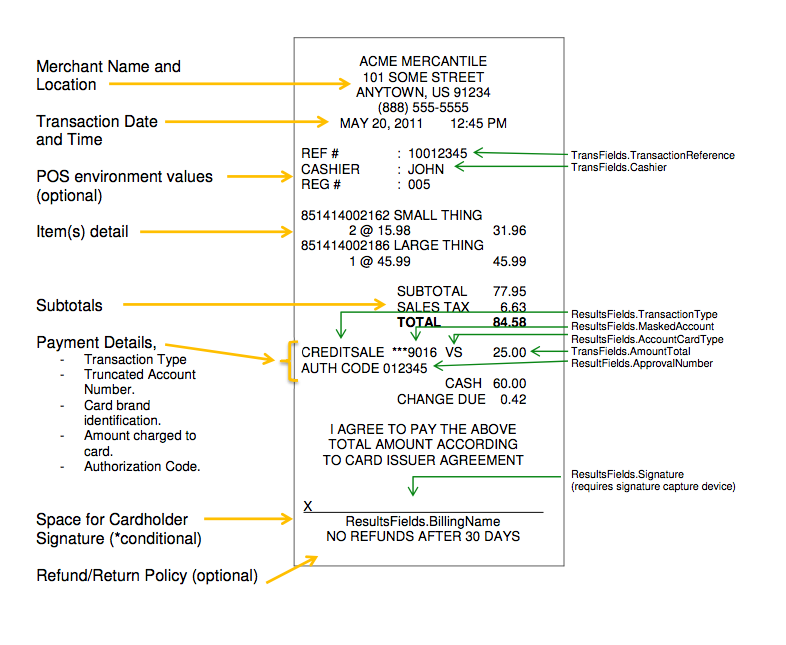
How To Process Customer Transactions
This is an example of a Customer Management Screen that has been integrated with EasyIntegrator.Note: The following grid contains only test data, so Deletes will just be local test data (i.e. you can't hurt anything).
Cust Ref | First Name | LastName | Phone | Zip | Last Transaction | ||
---|---|---|---|---|---|---|---|
{{customer.customerRef}} | {{customer.firstName}} | {{customer.lastName}} | {{customer.email}} | {{customer.phone}} | {{customer.zip}} | {{customer.lastTransaction}} | Add Customer |
Description
To use EasyIntegrator to process a customer transaction,
create a JSON object that contains a CustomerFields object
and pass it into EasyIntegrator.processTransaction.
Code Example
- var request = {
- "merchantName": "BobsRibs1",
- "merchantKey": "A3gziLB98002",
- "configurationId": "198e84701abc28da",
- "transactionType": "CreateCustomer",
- "customerFields": {
- "firstName": "Test",
- "lastName": "Customer",
- "address": "2 W 72nd St.",
- "address2": "",
- "city": "New York",
- "state": "NY",
- "zip": "10023"
- }
- };
- EasyIntegrator.processTransaction(request)
- .success(function (customer) {
- var customerRef = customer.customerRef;
- })
- .error(function (errorMessage) {
- alert(errorMessage);
- })
- .done(function () {
- });